Roles and Permissions
Learn about the Laravel Admin Panel permissions
The Laravel Admin Panel comes with Roles and Permissions. Each user has a role that has a set of permissions.
Inside the toolbar, you can add, edit, or delete the current roles. In addition, when you click to edit a specific role, you can specify CRUD permissions.
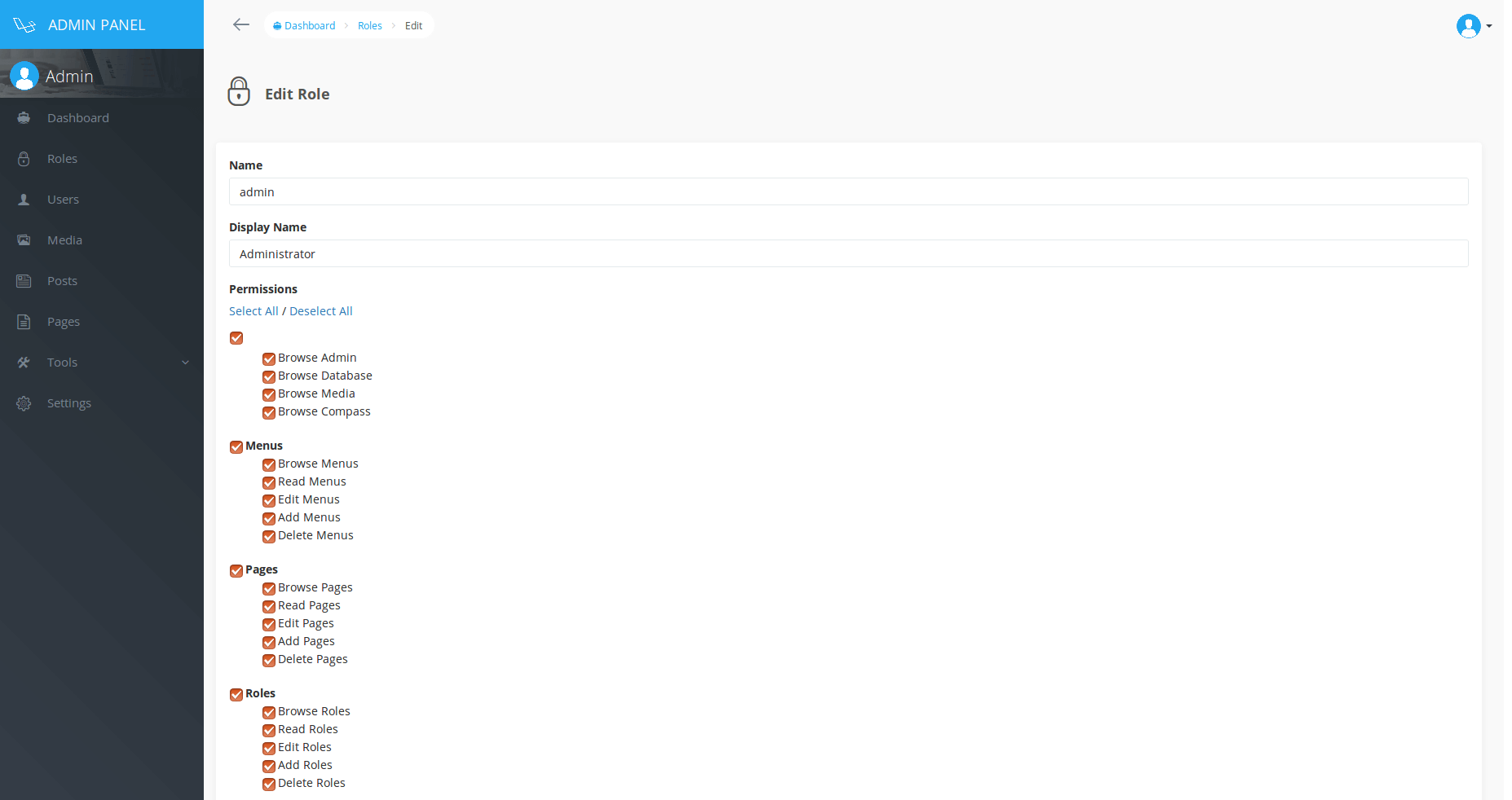
You can check for permissions in the following ways:
// via user object
$canViewPost = $user->can('read', $post);
$canViewPost = Auth::user()->can('read', $post);
// via controller
$canViewPost = $this->authorize('read', $post);
You can choose to use the Admin facade and pass the permission as a string:
$canBrowsePost = Admin::can('browse_posts');
$canViewPost = Admin::can('read_posts');
$canEditPost = Admin::can('edit_posts');
$canAddPost = Admin::can('add_posts');
$canDeletePost = Admin::can('delete_posts');
The value of each check returns a Boolean value if the user has a specific permission. However, you can refuse a prohibited exception if the user does not have a specific permission. This can be done using the canOrFail method:
Admin::canOrFail('browse_admin');
Out of the box there are some permissions you can use by default:
browse_admin
: Whether or not the user may browse the Laravel Admin Panel.browse_database
: Whether or not the user may browse the Laravel Admin Panel database menu section.browse_media
: Whether or not the user may browse the Laravel Admin Panel media section.browse_menu
: Whether or not the user may browse the Laravel Admin Panel menu section.browse_settings
: Whether or not the user may browse the Laravel Admin Panel settings section.read_settings
: Whether or not the user can view or see a particular setting.edit_settings
: Whether or not the user can edit a particular setting.add_settings
: Whether or not the user can add a new setting.delete_settings
: Whether or not the user can delete a particular setting.
Out of the box, there are some permissions that you can use by default:
browse_admin
: whether the user can view the Laravel Admin Panel.browse_database
: can the user view the database menu section of the Laravel Admin Panel.browse_media
: whether the user can view the section of the media section of the Laravel Admin Panel.browse_menu
: whether the user can view the menu section of the Laravel Admin Panel.browse_settings
: whether the user can view the settings section of theLaravel Admin Panel.read_settings
: whether the user can view or see a specific parameter.edit_settings
: whether the user can edit a specific setting.add_settings
: whether the user can add a new setting.delete_settings
: whether the user can delete a specific setting.
In addition, you can Generate Permissions
for each type of CRUD that you create. This will create browse
, read
, edit
, add
, and delete
permissions.
As an example, perhaps we are creating a new CRUD type from a products
table. If we choose to Generate permissions
for our products
table. Our permission keys will be browse_products
, read_products
, edit_products
, add_products
and delete_products
.
As an example, perhaps we are creating a new CRUD from the articles
table. If we select Generate permissions
for our articles
table. Our access keys will be browse_articles
, read_articles
, edit_articles
, add_articles
and delete_articles
.
Notice
If the menu item is associated with any kind of CRUD, then it will check the
browse
permission, for example, for the CRUD's 'articles' menu item, it will check thebrowse_articles
permission. If the user does not have the required permission, this menu item will be hidden.
Using Permissions in Blade Template files
You can also check permissions using the syntax of the blade. For example, that you want to check if a user can "browse_articles" is quite simply, we can use the following syntax:
@can('browse', $article)
I can browse articles
@else
I cannot browse articles
@endcan
Updated less than a minute ago